一、Ajax简介
Asynchronous JavaScript and XML(异步的 JavaScript 和 XML)。
不是新的编程语言,而是一种使用现有标准的新方法。
可以使网页实现异步更新,意味着在不重新加载整个页面的情况下,对网页的某部分进行更新。
不需要任何浏览器插件,但需要用户允许JavaScript在浏览器上执行。
注意:ajax本身不支持跨域请求,需要在服务器端处理。
二、Ajax 工作原理
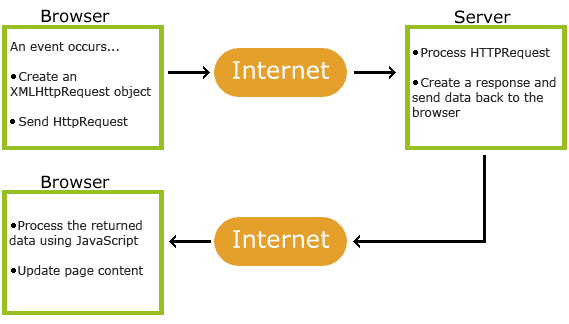
三、Ajax是基于现有的Internet标准
- XMLHttpRequest 对象 (异步的与服务器交换数据)
- JavaScript/DOM (信息显示/交互)
- CSS (给数据定义样式)
- XML (作为转换数据的格式)
AJAX应用程序与浏览器和平台无关的!
四、Ajax 使用步骤
创建ajax对象
1 | var xhr = null; |
连接服务器
1 | // 连接服务器open方法(GET/POST,请求地址, 异步传输) |
发送请求
1 | xhr.send(); |
接收返回数据
1 | /* |
五、GET 请求
1 |
|
六、POST 请求
1 |
|
七、url - 服务器上的文件
open() 方法的 url 参数是服务器上文件的地址:
1 | xmlhttp.open("GET","ajax_test.html",true); |
该文件可以是任何类型的文件,比如 .txt 和 .xml,或者服务器脚本文件,比如 .asp 和 .php (在传回响应之前,能够在服务器上执行任务)。
八、onreadystatechange 事件
当请求被发送到服务器时,我们需要执行一些基于响应的任务。
每当 readyState 改变时,就会触发 onreadystatechange 事件。
readyState 属性存有 XMLHttpRequest 的状态信息。
下面是 XMLHttpRequest 对象的三个重要的属性:
onreadystatechange
存储函数(或函数名),每当 readyState 属性改变时,就会调用该函数。
readyState
- 0: 请求未初始化
- 1: 服务器连接已建立
- 2: 请求已接收
- 3: 请求处理中
- 4: 请求已完成,且响应已就绪
status
- 200: “OK”
- 404: 未找到页面
当 readyState 等于 4 且状态为 200 时,表示响应已就绪:
onreadystatechange 事件被触发 4 次(0 - 4), 分别是: 0-1、1-2、2-3、3-4,对应着 readyState 的每个变化。
九、封装使用
1 |
|